Define the cell type as value using an office script in an excel report
Coming from macro, I got confused about the use of the cell value for a script at the beginning. In macro, it is quite simple as you know, for instance, I have this value in B1:
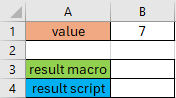
And with the VBA, I want to sum it with another value like that:
Sub test() Dim mainvalue mainvalue = Range("B1") Range("B3") = mainvalue + 9 End Sub
The result is:

If I do the same logic for the script:
function main(workbook: ExcelScript.Workbook) { let mainvalue = workbook.getActiveWorksheet().getRange("B1").getValue(); workbook.getActiveWorksheet().getRange("B4").setValue(5 + mainvalue); }
It will work but in the code, I will get a red highlight as an error:
![]() |
![]() |
To avoid it, I have to define the cell type. In Office script, when I am using a cell value, it's important to tell the script what value I am expecting to get from a cell or range. A cell contains one of the following types:
- string: a sequence of one or more characters that may consist of letters, numbers, or symbols
- number: a numerical value
- Or boolean: a result that can be defined as TRUE or FALSE
In order for my script to treat the returned values as one of those types, I must declare the type. For my example, it will be like that:
function main(workbook: ExcelScript.Workbook) { let mainvalue = workbook.getActiveWorksheet().getRange("B1").getValue() as number; workbook.getActiveWorksheet().getRange("B4").setValue(5 + mainvalue); }
NOTE: I added “as number” at the end of “mainvalue”
Don’t mix up with “as String”, “as Number” or “as Boolean” with a capital letter which are treated as an “interface” versus “as string”, “as number” or “as boolean” without a capital letter which are treated as a “value”.
An interface allows to define the expected structure of an object providing a way to describe the shape of objects, including their properties and methods, without implementing any functionality.
To resume, based on what I want to do and for my script to work correctly, I don’t have to forget to define the cell as string, number or boolean.
Interesting Topics
-
Be successfully certified ITIL 4 Managing Professional
Study, study and study, I couldn’t be successfully certified without studying it, if you are interested...
-
Be successfully certified ITIL 4 Strategic Leader
With my ITIL 4 Managing Professional certification (ITIL MP) in the pocket, it was time to go for the...
-
Hide visual and change background color based on selection
Some small tricks to customize the background colour of a text box...
-
Stacked and clustered column chart or double stacked column chart
In excel, I use a lot the combination of clustered and stacked chart...
-
Refresh Power BI
From the Power BI Service, I can set refresh but, for instance, there is no option to do it monthly or each time a change is made...
-
Power BI alerts to be sent by email from an excel file based on condition
I will explain how to send a list of emails from an excel file after creating alerts...